How to reverse a string in Python
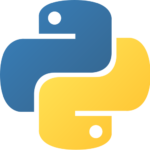
Reversing all the letters of a string is a basic coding exercice. But Python does have its special tools and the standard algorithm isn’t the one to choose for this language. Python does have his way to make things easier. And this reason is odd for a beginner.
So, lets see in this post what are the constraints to work with string in Python and how Python makes things easier.
Strings and Python idioms
Strings are immutable in Python. You can’t modify a string. This means that you can’t add, delete one or replace a character. So, there is no reverse() method as for lists. Remember that the reverse() method of lists does not return anything, which means that the original list is modified.
In [1]camelot = ["Arthur", "Lancelot", "Robin"] In [2]: camelot.reverse() In [3]: camelot Out[3]: ['Robin', 'Lancelot', 'Arthur']
Should the same method exist for strings, the same behavior would be expected. Which is impossible.
The reversed() function
In Python, there is a built-in function named reversed(seq). This function takes one parameter which must be a sequence. The function returns an iterator on the reversed of that sequence:
In [1]: reversed("abc") Out[1]: <reversed at 0x104ff97d0>
Strings are sequences, so, to get the reversed string, you could write the following code:
In [2]: "".join(reversed("abc")) Out[2]: 'cba'
And what about the slicing ?
Slicing is a very powerful tool in Python and can be used with any sequence type. Don’t forget that slicing takes up to 3 parameters, the third is the step which can be negative. So, we can write:
In [3]: "abc"[::-1] Out[3]: 'cba'
This is really the shortest instruction I can write.
NEVER do the following
Really, please, don’t write this kind of function:
In [1]: def miror(seq): ...: rseq = "" ...: for char in seq: ...: rseq = char + rseq ...: return rseq ...: In [2]: miror("abc") Out[2]: 'cba'
Yes, it does the job. The Web is full of this kind of code. But this code is bad for several reasons.
First, remember that in Python, variables are references to the values. On each iteration, a new string is created and the rseq variable changes it references. This has its cost.
The second reason is about readability. You have to read 4 lines to understand the purpose of this code. Readability counts, think about those who will have to read your code.
The third reason deserves its own section…
What about execution time ?
Let’s check the execution time for all of those methods.
In [1]: %timeit "".join(reversed("abs")) 397 ns ± 5.7 ns per loop (mean ± std. dev. of 7 runs, 1000000 loops each) In [2]: %timeit "abc"[::-1] 112 ns ± 0.763 ns per loop (mean ± std. dev. of 7 runs, 10000000 loops each) In [3]: %timeit miror("abc") 390 ns ± 66.3 ns per loop (mean ± std. dev. of 7 runs, 1000000 loops each)
Do you really need any comment ? Slicing is definitely the most efficient way to reverse a string: shorter code and fastest to execute.
So, next time you are looking to reverse a string in Python, remember the tools provided by the language.